C3.1 Solve problems and create computational representations of mathematical situations by writing and executing code, including code that involves conditional statements and other control structures.
Skill: Computational Problem Solving
Coding can be used to automate tasks and to represent and solve problems. By its very nature, coding lends itself well to trial-and-error learning, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "What will happen if…?".
In addition, the question "what will happen if…?" allows one to start a conversation about conditions and conditional statements. For example, in a probability problem, one might ask "what will happen if I roll a die 1,000 times?" or even "how many times will I get 2 by rolling a die 1,000 times?" Given the time required to perform this experiment manually, code becomes an efficient way to solve such a problem.
Skill: Representing Mathematical Situations Computationally
Coding can be used as a mathematical learning tool to represent and manipulate complex mathematical situations.
For example, the representation of the die problem can be done with a code sequence that looks like this:
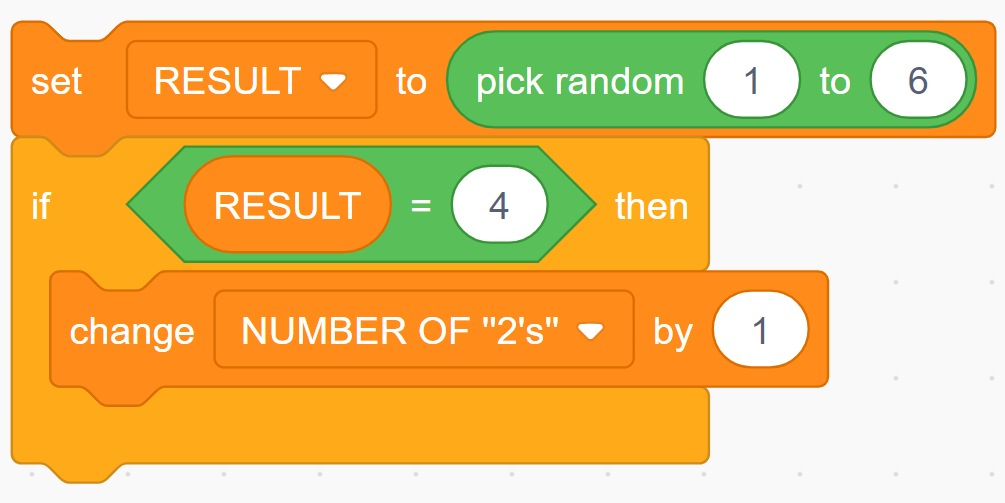
In this code, a random number from 1 to 6 is used to represent the action of rolling a die, and the conditional statement "if result = 2, then add 1 to the variable (number of "2")" essentially counts the number of times the number 2 is rolled.
The student could also associate a conditional instruction with a sprite to visually represent the faces of the die depending on the outcome.
Skill: Writing Code
Writing and altering a code involves sequencing instructions in a specific order, following the syntax of a programming language. Writing code can be similar to writing text. Pseudocode is writing the instructions for a code in no particular program language.
With the addition of conditional statements, for example, the student has several commands at his disposal, which makes it possible to make more complex codes. However, this requires the use of a certain syntax when writing the pseudocode. The student will first need to use spaces and indents strategically to represent the intent of the pseudocode and to facilitate transfer to code.
Here is an example of pseudocode in which suits are associated with the result obtained after rolling a die. Here, we use the "if, then, else" command to allow several possibilities.
Sprite - DIE Costumes: Variables: RESULT |
SET "RESULT" TO (RANDOM NUMBER 1-6) |
IF "RESULT" = 1 THEN PUT ON COSTUME: OR |
IF "RESULT" = 2 THEN PUT ON COSTUME: OR |
IF "RESULT" = 3 THEN PUT ON COSTUME: OR |
IF "RESULT" = 4 THEN PUT ON COSTUME: OR |
IF "RESULT" = 5 THEN PUT ON COSTUME: OR PUT ON COSTUME: |
By placing the statements (if, then, else) on a new line and indented, it will be easier to transform this pseudocode into code on the computer. Note that the pseudocode is intended to make it easier for the student to understand the code, so the exact structure may differ from student to student. The important thing is that the student can understand what is going on in the pseudocode.
Skill: Executing Code
Execution of code is when the code is read and compiled by the coding program and is referred to as the output. In block coding, the execution of a code is often done by means of a button in the interface, while text-based programming languages require precise compilation program that essentially translates the code from the programming language to the machine language (for example, binary code) so that it can execute the code. This also applies to robotic devices.
Some examples of run buttons for block coding program

Source: makecode.
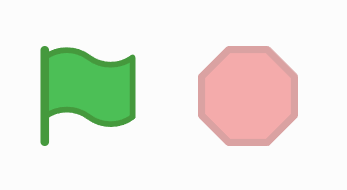
Source: Scratch Jr.
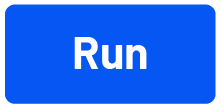
Source: Programiz.
Knowledge: Conditional Instructions
A conditional statement is a type of coding command that instructs the program to compare values and expressions and then make decisions based on whether the condition is true or false.
Conditional statements are first present when the execution of an event or a sequence of code depends on the achievement of a specific condition. Boolean values (true or false) are often used and associated with terms such as if, else, until and when.
The code allows the representation of multiplications using the rectangular layout and could be transformed to determine whether the layout represents a square or not. It would then be the following pseudocode:
If "value_1" = "value_2
So
Say "Yes, it's a square!"
Otherwise
Saying "No, it's not a square!"
Since we are talking about a geometric shape, the names given to "value_1" and "value_2" could be more precise, like "base" and "height". Here is a representative code example of this pseudocode:
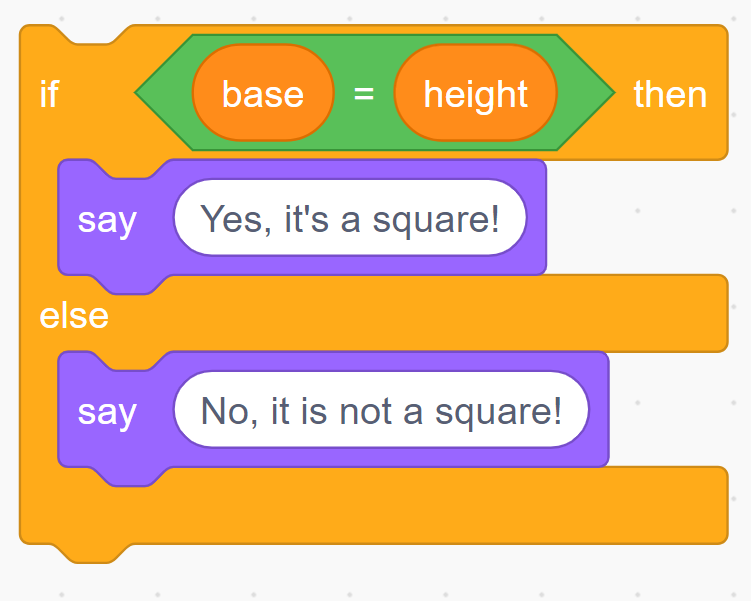
Knowledge: Events and Structures (Prior Knowledge)
Sequential Events
A set of instructions executed one after the other.
Example

Simultaneous Events
Several events that occur at the same time.
Example
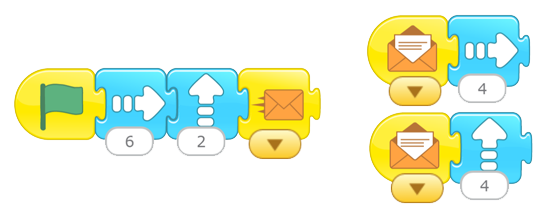
Recurring Events
Repeating events. In coding activities, loops are used in code to repeat instructions.
Example
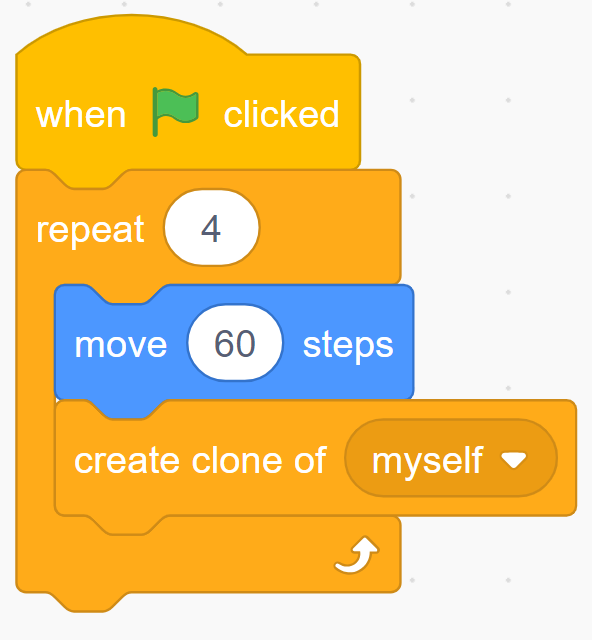
Nested Events
Control structures placed inside other control structures. For example, loops occurring inside loops or a conditional statement being evaluated inside a loop.
Example
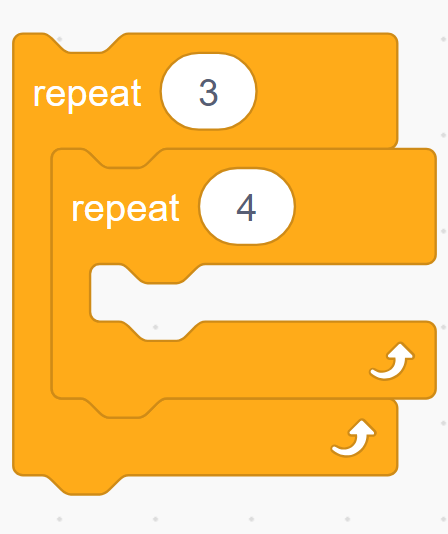